Negative Transformation:
Image inversion or Image negation helps find the details from the darker regions of the image. The negative of an image is achieved by replacing the intensity āiā in the original image with āi-1ā, i.e. the darkest pixels will become the brightest and the brightest pixels will become the darkest. Image negative is produced by subtracting each pixel from the maximum intensity value.
For instance, in an 8-bit grayscale image, the max intensity value is 255, thus each pixel is subtracted from 255 to produce the output image.
function Negative(Name)
img=imread(Name);
img=img(:,:,1);
m=length(img(:,1));
n=length(img(1,:));
for i=1:m
for j=1:n
img(i,j)=255-img(i,j);
end
end
imshow(img)
title('Negative image')
end
Log transformation
Log transformation is used for image enhancement as it expands dark pixels of the image as compared to higher pixel values. In this transformation, we replace each pixel value with its logarithm. In the following code, the value of “c” is chosen such that we get the maximum output value corresponding to the bit size used. For instance, for an 8-bit image, “c” is chosen such that we get a maximum value of 255.
function LogTransform(Name,c)
img=imread(Name);
img=img(:,:,1);
m=length(img(:,1));
n=length(img(1,:));
for i=1:m
for j=1:n
img(i,j)=c*log(1+double(img(i,j)));
end
end
imshow(img)
title('Log transform image')
end
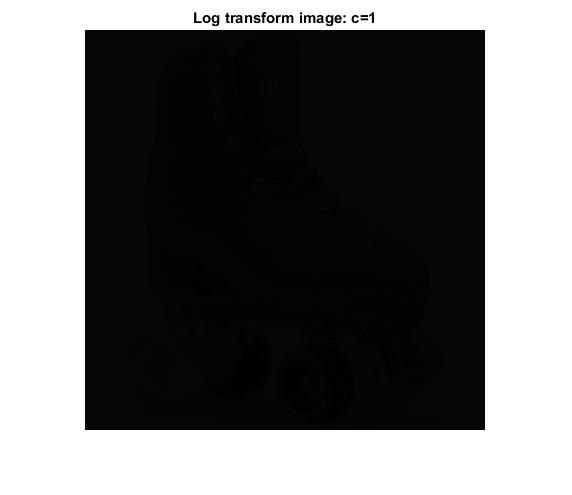
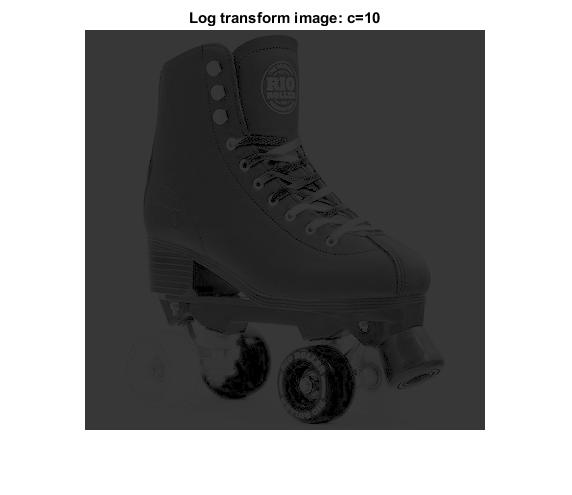
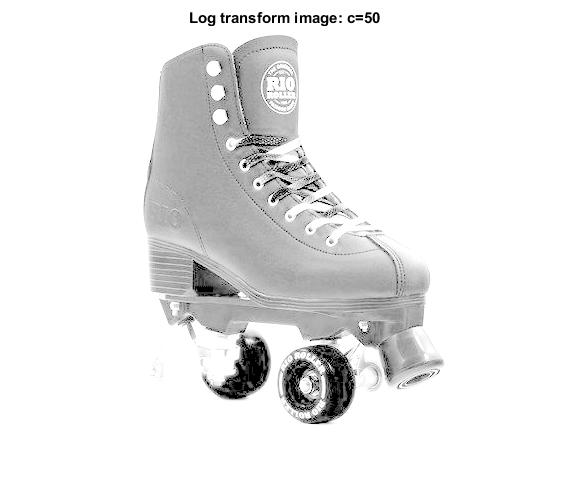
Power law transformation
function Power_low(Name,c,gamma)
img=imread(Name);
img=img(:,:,1);
m=length(img(:,1));
n=length(img(1,:));
for i=1:m
for j=1:n
img(i,j)=c*double(img(i,j))^gamma;
end
end
imshow(img)
title('Power-low transform image')
end
end
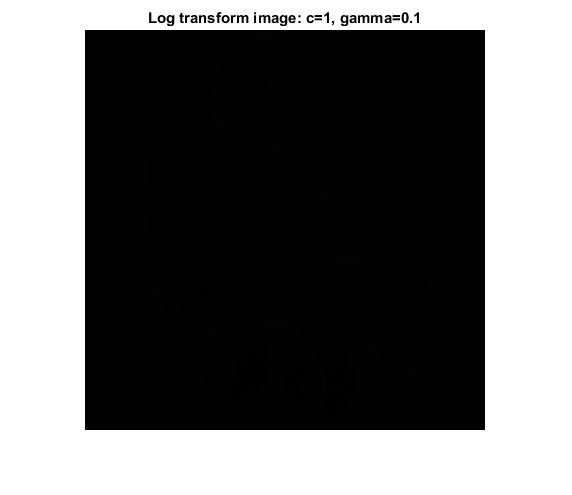
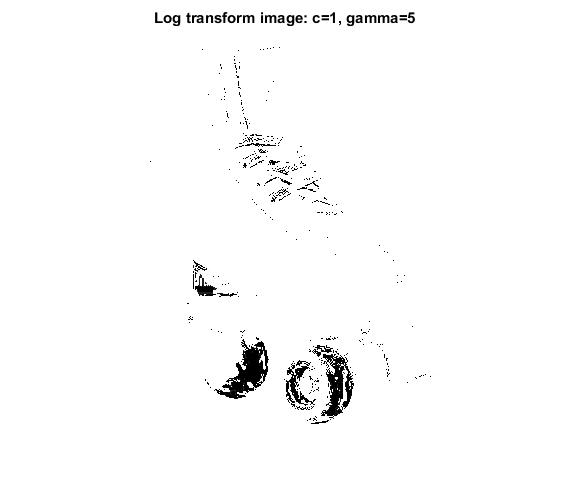
Linear segment transformation
function LinearStrech(Name,r_low,r_high,r_min,r_max)
img=imread(Name);
img=img(:,:,1);
m=length(img(:,1));
n=length(img(1,:));
for i=1:m
for j=1:n
if img(i,j)<=r_low
rlow_temp=0;
rhigh_temp=r_low;
rmin_temp=0;
rmax_temp=r_min;
img(i,j)=rmin_temp+(img(i,j)-rlow_temp)*(rmax_temp-rmin_temp)/(rhigh_temp-rlow_temp);
elseif img(i,j)>r_low && img(i,j)<=r_high
img(i,j)=r_min+(img(i,j)-r_low)*(r_max-r_min)/(r_high-r_low);
elseif img(i,j)>r_high
rlow_temp=r_high;
rhigh_temp=255;
rmin_temp=r_max;
rmax_temp=255;
img(i,j)=rmin_temp+(img(i,j)-rlow_temp)*(rmax_temp-rmin_temp)/(rhigh_temp-rlow_temp);
end
end
imshow(img)
title('Linear contrast stretching transform image')
end